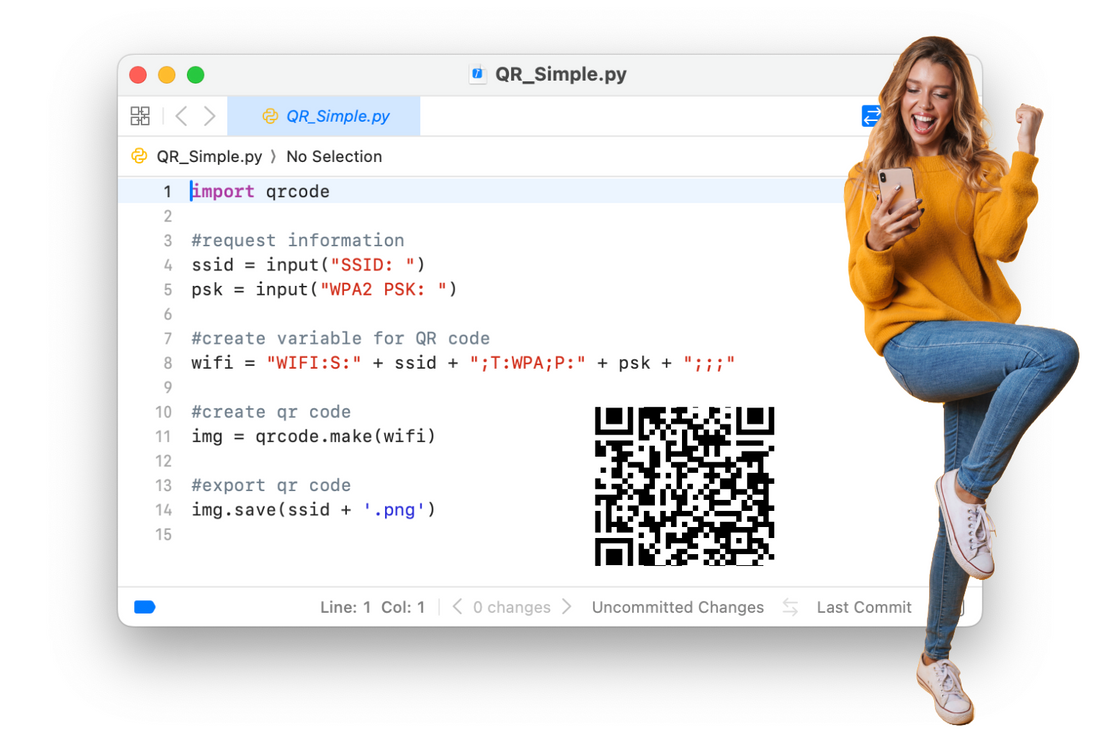
Create your own QR code for easy WLAN login - with Python
Share
Introduction
How many of you have used a QR code to connect to a Wi-Fi?
We often come across this option on public networks or in companies. Of course, this is usually only intended for guests - I'd rather protect my internal WLAN with secure authentication.
A QR code is also often printed on home Wi-Fi routers. However, you should definitely change the SSID and password here, as the QR code will of course no longer work afterwards.
Generate WLAN QR code yourself
So how can I generate a QR code for my WLAN myself?
Online without effort
A quick Google search provides numerous free online generators. But who wants to enter their Wi-Fi password on someone else's website?
“It's just a simple password that I don't use anywhere else”, you might think. And how are they supposed to know where my Wi-Fi is available?
In fact, you not only enter your password, but also your SSID. Although this often does not contain any unique information about your location, services such as WiGLE collect the locations of SSIDs worldwide.
If your SSID is unique, there is a good chance that you will be able to find it on WiGLE. On the other hand, do you have a generic SSID like “Guest”? Then others might not be able to connect. Anyone who has already connected with an identical SSID and a different password will automatically try with this data. Unsuccessfully, of course!
So how can I create a QR code safely and easily?
Create QR codes locally and offline
It's easy - with Python! Python is used by many developers and technicians and is already pre-installed on macOS. What we are still missing is the QRCode library.
You can easily install this library via the terminal:
pip3 install qrcode
You can then use the following script to generate your own QR code.
import qrcode
# Benutzereingaben
ssid = input("SSID: ")
psk = input("WPA2 PSK: ")
# QR-Code-Daten erstellen
wifi = "WIFI:S:" + ssid + ";T:WPA;P:" + psk + ";;;"
# QR-Code generieren
img = qrcode.make(wifi)
# QR-Code speichern
img.save(ssid + '.png')
The result
A QR code in PNG format that you can now use to connect to your Wi-Fi.
Important note: The password is stored in plain text in the QR code. The QR code therefore does not protect your password from being accessed by others.
Colorful barcode?
Of course, the Python script can still be revised. With the following Python code you can customize the QR code. There are three parameters:
- error_correction
- Error correction, this can be used to adjust the redundancy of the barcode. A higher error correction ensures better readability, but also larger barcodes.
- Possible parameters:
- _L for 7%
- _M for 15%
- _Q for 25%
- _H for 30%
- box_size
- This parameter can be used to adjust the size of the individual boxes in the QR code.
- border
- This parameter can be used to adjust the size of the white border around the QR barcode.
The color of the QR code and background can then be adjusted during creation. To make the barcode legible, there should be a certain contrast between the background and the QR code.
import qrcode
#request information
ssid = input("SSID: ")
psk = input("WPA2 PSK: ")
#create variable for QR code
wifi = "WIFI:S:" + ssid + ";T:WPA;P:" + psk + ";;;"
#create qr code object
qr_object = qrcode.QRCode(
version=1,
error_correction=qrcode.ERROR_CORRECT_H, #change error correction _L 7% _M 15% _Q 25% _H 30%
box_size=10, #change size of the QR code boxs def. 10
border=10, #change size of the border around the QR code def. 4
)
qr_object.add_data(wifi)
qr_object.make(fit=True)
img = qr_object.make_image(fill_color="green", back_color="blue")
img.save(ssid + '.png')
The choice of colors should perhaps be a little more considered than in my example.